Facebook Ads Insights Api Python
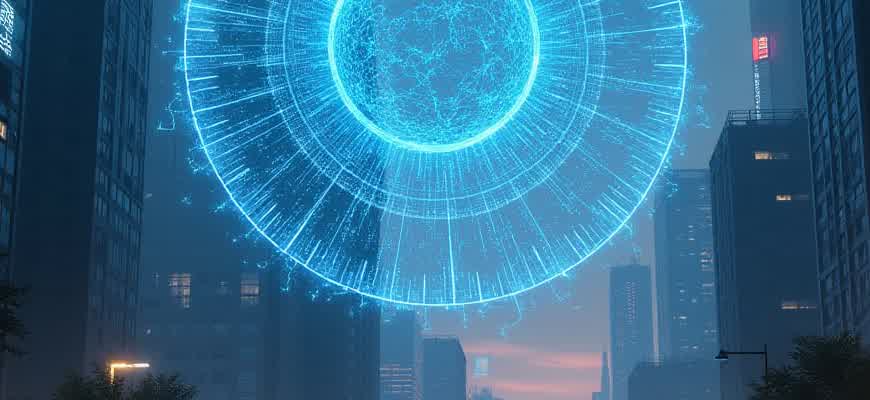
Facebook Ads Insights API offers a powerful way to access and analyze advertising data. By leveraging Python, marketers can automate the extraction and processing of detailed campaign metrics. This allows for in-depth performance analysis and better decision-making for ad strategies.
To begin, you need to set up the necessary environment for accessing the Facebook Ads Insights API using Python. Follow these steps:
- Create a Facebook Developer account and set up an App.
- Obtain an Access Token with appropriate permissions.
- Install Python packages such as requests or facebook-business.
- Set up API queries to extract insights data for your ads.
Once the environment is ready, you can interact with various endpoints to retrieve key metrics such as impressions, clicks, and conversions.
Important: Always ensure that your Access Token is kept secure and regularly refreshed to prevent unauthorized access to your data.
Here is an example of how you can use Python to make API requests:
import requests url = 'https://graph.facebook.com/v12.0/{ad_account_id}/insights' params = { 'access_token': 'your_access_token', 'fields': 'campaign_name,impressions,clicks,spend', 'time_range': {'since':'2021-01-01', 'until':'2021-12-31'} } response = requests.get(url, params=params) data = response.json()
By using this approach, you can begin extracting relevant ad insights in a structured format for further analysis.
Facebook Ads Insights API Python: Practical Guide
The Facebook Ads Insights API is a powerful tool for fetching performance data from Facebook advertising campaigns. Using Python, developers can automate the retrieval and analysis of ad metrics, providing actionable insights to optimize ad spend and targeting. By leveraging this API, businesses can easily track key performance indicators (KPIs) such as impressions, clicks, conversions, and much more in real-time.
In this guide, we will walk through the essential steps for accessing Facebook Ads Insights using Python. From setting up access to making API calls, we’ll break down the process in a way that’s both practical and easy to follow. With a solid understanding of the API’s capabilities, you can implement customized reports and automate data analysis to drive better advertising strategies.
Key Steps to Get Started with the API
- Set up a Facebook Developer account and create an app.
- Obtain the necessary credentials (Access Token, App ID, etc.).
- Install the required Python libraries, such as 'requests' or 'facebook-business'.
- Make API calls to the Ads Insights endpoint and retrieve data.
Example of a Basic API Call in Python
Here’s how to make a simple API call to fetch the insights data for your Facebook ads campaigns:
import requests access_token = 'your_access_token' ad_account_id = 'your_ad_account_id' url = f'https://graph.facebook.com/v12.0/{ad_account_id}/insights' params = { 'access_token': access_token, 'fields': 'campaign_name,impressions,clicks,spend', 'date_preset': 'last_30_days' } response = requests.get(url, params=params) data = response.json() print(data)
Understanding the Data
After making the API call, you will receive data in a JSON format. Below is an example of the key metrics you might encounter:
Metric | Description |
---|---|
campaign_name | Name of the campaign |
impressions | The number of times the ad was shown |
clicks | The number of clicks on the ad |
spend | The total amount spent on the campaign |
Important: Make sure to handle pagination if you’re fetching a large amount of data. The API will return results in pages, and you need to implement logic to handle subsequent pages using the "next" field in the response.
Setting Up Your Facebook Ads Insights API in Python
Integrating the Facebook Ads Insights API with Python allows you to access and analyze ad performance data in real-time. To begin, you'll need to set up the necessary environment, install the required libraries, and authenticate your API requests. This process can seem overwhelming at first, but breaking it down into simple steps makes it manageable.
The setup begins with obtaining the necessary credentials from Facebook's Developer portal, followed by installing the Python SDK. Once you have access to the API and the necessary tools, you can start pulling valuable insights into your ad campaigns.
Steps to Set Up the API
- Create a Facebook Developer Account: Go to the Facebook Developer Portal and sign up for an account.
- Create an App: After signing in, create a new app under your Developer account to generate an App ID and App Secret.
- Obtain Access Tokens: Use the App ID and App Secret to obtain a long-lived access token that allows access to your Facebook Ads data.
- Install Required Libraries: Use Python’s
pip
to install the necessary SDK:pip install facebook-business
- Authenticate Your API Requests: Use the access token for API authentication in your Python code.
Important Setup Information
Ensure that the Facebook Ads API is enabled for your account before proceeding with the setup. Without this, you won’t be able to pull data from your ads.
Sample Code to Get Started
Here’s a basic example of how to connect to the API and fetch campaign data:
from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.adaccount import AdAccount access_token = 'your_access_token' ad_account_id = 'act_your_ad_account_id' FacebookAdsApi.init(access_token=access_token) account = AdAccount(ad_account_id) campaigns = account.get_campaigns() for campaign in campaigns: print(campaign)
Handling API Limits
When working with the Facebook Ads API, it’s essential to keep track of your usage limits to avoid hitting API rate limits:
Limit Type | Value |
---|---|
Rate Limit | 200 requests per hour |
Data Limit | 1000 rows per query |
Understanding API Authentication for Facebook Ads with Python
When working with the Facebook Ads Insights API, authentication is a crucial step before making any API calls. It ensures secure access to your ad account data while safeguarding sensitive information. The most common method of authentication for the Facebook API is OAuth 2.0, which is widely used for ensuring secure access to resources in web applications.
In this context, the first step is to obtain an access token, which acts as a key to authenticate your requests. Facebook’s platform uses two types of tokens: the User Access Token and the App Access Token. Both have different levels of permissions and are used based on your needs, but for interacting with Ads Insights, the User Access Token is most commonly required.
Authentication Process
- Register your app in the Facebook Developers portal.
- Generate an App ID and App Secret.
- Request permission from the user to access their ad account data.
- Obtain an access token using OAuth 2.0.
Access Token Types
- User Access Token: This token is obtained after the user grants permission. It allows access to specific ad account data tied to the user.
- App Access Token: This token allows the app itself to access its own resources, but is limited to the app's scope.
Note: Access tokens can expire, so it's essential to refresh them periodically to avoid interruptions in your API interactions.
Example Authentication Flow
Step | Action |
---|---|
1 | Direct the user to Facebook’s OAuth URL. |
2 | Allow the user to authenticate and grant permissions. |
3 | Obtain the authorization code, then exchange it for an access token. |
4 | Use the access token to make API requests to the Ads Insights API. |
How to Retrieve Campaign Metrics Using Facebook Ads Insights API
When working with Facebook Ads Insights API, retrieving campaign data is a key task for advertisers seeking to analyze the performance of their ad campaigns. The process involves making API requests to gather data on various metrics like impressions, clicks, conversions, and more. This data helps in evaluating the effectiveness of campaigns and optimizing future strategies.
To fetch campaign data, you will need to interact with the Ads Insights endpoint, providing the necessary parameters to specify the campaign you want data for. Here's a breakdown of the steps involved:
Steps to Fetch Campaign Data
- Authenticate via Facebook's Graph API using an access token that has permission to access Ads Insights data.
- Make a request to the `/insights` endpoint, providing the campaign ID and specifying the fields you want to retrieve.
- Process the returned data, which includes metrics such as reach, engagement, and ad spend for the specified campaign.
Here is an example of how to make an API request using Python:
import requests url = 'https://graph.facebook.com/v15.0/{campaign_id}/insights' params = { 'access_token': '{your_access_token}', 'fields': 'campaign_name,impressions,clicks,spend,reach', 'date_preset': 'last_30_days' } response = requests.get(url, params=params) data = response.json() print(data)
Important: Ensure your access token has the appropriate permissions and that the campaign ID is correct for accurate data retrieval.
Understanding the Response
The response will contain a JSON object with an array of data for each campaign. The data is structured as follows:
Field | Description |
---|---|
campaign_name | The name of the campaign. |
impressions | The total number of times the ad was shown to users. |
clicks | The total number of clicks the ad received. |
spend | The total amount of money spent on the campaign. |
reach | The number of unique users who saw the ad. |
With this approach, you can gather the essential metrics to assess your campaign's performance and make informed decisions about future ad strategies.
Analyzing Key Metrics from Facebook Ads Insights with Python
To effectively assess the performance of Facebook ads, it is essential to extract and analyze key metrics from the Facebook Ads Insights API using Python. This process enables marketers to evaluate campaign efficiency and optimize ad strategies in real time. Python's flexibility and rich libraries make it an excellent choice for automating data extraction, processing, and visualization tasks from the Facebook platform.
With the proper setup of the Facebook Marketing API and Python’s data analysis libraries such as Pandas and Matplotlib, it becomes easier to retrieve and interpret data such as impressions, reach, click-through rates, and more. The following sections break down essential metrics and ways to analyze them using Python.
Key Metrics to Track
Here are some of the most important metrics to monitor when analyzing Facebook ads:
- Impressions - The total number of times the ad was displayed.
- Reach - The unique number of people who saw the ad.
- Click-through rate (CTR) - The ratio of clicks to impressions, reflecting ad effectiveness.
- Cost per click (CPC) - The amount spent per user click on the ad.
- Return on Ad Spend (ROAS) - The revenue generated for every dollar spent on the campaign.
Using Python for Data Analysis
After retrieving data from the Facebook Ads Insights API, the next step is to analyze it using Python. Below is a general approach:
- Set Up the API: Use the Facebook SDK for Python to authenticate and fetch ad performance data.
- Data Parsing: Convert the raw data into a format that can be manipulated with libraries like Pandas.
- Analysis: Calculate and visualize metrics such as CPC, CTR, and ROAS to evaluate the effectiveness of ads.
- Visualization: Use Matplotlib or Seaborn to create visualizations like bar charts or line graphs for clearer insights.
Tip: Use Pandas DataFrames to efficiently filter, aggregate, and compute metrics from large sets of ad data.
Example Metrics Table
Below is an example of how ad metrics can be structured into a table for easier analysis:
Ad ID | Impressions | Reach | CTR (%) | CPC | ROAS |
---|---|---|---|---|---|
12345 | 500,000 | 400,000 | 3.5 | $0.50 | 4.2 |
67890 | 600,000 | 550,000 | 2.8 | $0.75 | 3.5 |
Building Custom Reports from Facebook Ads Insights Data
Creating custom reports based on Facebook Ads Insights allows marketers to tailor data visualization and analysis according to specific business needs. By leveraging the API, you can extract key performance indicators (KPIs) such as impressions, clicks, and conversions, and analyze them for various timeframes, campaigns, and ad sets. This flexibility enables businesses to gain actionable insights that are crucial for campaign optimization.
When building custom reports, the ability to filter and segment data effectively is essential. You can choose from a wide range of metrics and dimensions to define the report parameters, ensuring that the data generated is both relevant and easy to interpret. This process can help identify trends, monitor ad performance, and make informed decisions about budget allocation and targeting strategies.
Steps to Create a Custom Report
- Define the metrics: Choose the relevant KPIs such as reach, click-through rate (CTR), conversion rate, or return on ad spend (ROAS).
- Select the time frame: Determine the reporting period (daily, weekly, or custom dates) based on your campaign goals.
- Segment the data: Filter by campaign, ad set, or individual ads to analyze performance at different levels.
- Set the granularity: Choose whether to display data on a daily, weekly, or monthly basis.
- Export the results: Download the report in CSV or JSON format for further analysis or integration with other tools.
Example Report Structure
Metric | Value |
---|---|
Impressions | 150,000 |
Clicks | 2,500 |
CTR | 1.67% |
Conversions | 250 |
Cost per Conversion | $10 |
Note: Always ensure that the data retrieved is aligned with the business goals and adjusted for any seasonal fluctuations that might affect performance.
Key Takeaways
- Custom reports can provide deep insights into ad performance across different segments.
- Using the API for custom reports ensures real-time access to data and the ability to track ongoing campaign performance.
- Fine-tuning report filters and segments will allow you to uncover actionable insights and optimize your campaigns.
Handling Errors and Debugging in Facebook Ads API Requests
When working with the Facebook Ads API, encountering errors is an inevitable part of the development process. Understanding how to handle and debug these issues effectively can save time and ensure smooth integration. Errors may arise from incorrect parameters, authentication issues, or limitations imposed by Facebook's rate limits. Handling these issues promptly requires using proper error-catching techniques and interpreting the messages returned by the API.
When debugging API requests, it's crucial to analyze the response code and the error message to pinpoint the problem. The API will typically provide a response in JSON format, containing both the status and details about the error. Developers must understand how to interpret these responses to fix the issue quickly and prevent recurring errors in the future.
Error Handling Strategies
There are several key strategies to manage errors when working with Facebook's Ads API:
- Check the HTTP Status Code: Always verify the response's HTTP status code. A code of 200 indicates success, while 400 and 500 series codes point to client or server errors, respectively.
- Use Try-Except Blocks: For Python developers, implementing try-except blocks around the API call can help catch exceptions and handle them without crashing the program.
- Log Errors: Maintain detailed logs of any errors or failed requests. This practice will help in understanding the context in which the error occurred and provide insights for debugging.
Common Errors and Solutions
Understanding common API errors and their corresponding fixes is crucial for efficient troubleshooting:
- Invalid Permissions: Ensure the access token has the appropriate permissions to access the requested data.
- Missing Parameters: Double-check that all required fields are included in the request. For instance, forgetting to specify a campaign ID can result in a 400 error.
- Rate Limits Exceeded: Facebook enforces rate limits on API calls. If exceeded, the API will return a 429 status code. You can either retry after the timeout or optimize your requests to reduce frequency.
Inspecting API Responses
To effectively debug, examine the JSON response for error details. The response will typically include an error code, message, and type. Here's an example of a typical response structure:
Error Code | Message | Type |
---|---|---|
100 | Invalid parameter | OAuthException |
102 | Access token expired | AuthenticationError |
Tip: Always refer to the official Facebook API documentation for the most up-to-date error codes and descriptions to better understand and resolve issues.
Automating Data Retrieval for Consistent Insights Updates Using Python
Regular data updates from Facebook's Ads Insights API can be time-consuming without automation. Automating the retrieval process ensures that businesses always have access to the latest performance metrics, saving both time and resources. By leveraging Python's capabilities, you can set up scripts that retrieve and process the necessary data at specified intervals. This approach also eliminates human error and manual delays, providing more consistent and timely insights for decision-making.
Implementing automation is straightforward and can be done through the integration of the Facebook Graph API with Python libraries like `requests`, `pandas`, and `schedule`. These libraries help streamline the process of fetching, organizing, and storing data for ongoing analysis. With minimal setup, you can automate the process and ensure continuous monitoring of advertising campaigns without needing to constantly re-run the queries manually.
Steps for Automating Data Retrieval
- Authentication and Access: First, ensure that the application has access to the Facebook Ads Insights API using the correct access token. This is the foundation for making authenticated requests.
- Scheduling Retrieval: Use the `schedule` library to set up automatic queries at desired intervals, such as hourly or daily. This allows you to regularly fetch fresh data.
- Data Processing: Utilize `pandas` to organize and clean the retrieved data. This enables easy storage in databases or CSV files for future analysis.
- Integration with Alerts: You can set up email notifications or alerts to notify you if specific campaign thresholds are met or exceeded.
Example of a Data Retrieval Script
import requests import pandas as pd # Define the API endpoint and parameters url = 'https://graph.facebook.com/v11.0/act_{ad_account_id}/insights' params = { 'access_token': 'your_access_token', 'date_preset': 'last_30_days', 'fields': 'campaign_name,impressions,clicks,spend' } # Send the request and get the response response = requests.get(url, params=params) data = response.json() # Convert to DataFrame for analysis df = pd.DataFrame(data['data']) print(df)
Note: Ensure your API access token is kept secure and never hard-coded in production environments.
Key Benefits of Automating Insights Retrieval
- Efficiency: Automation reduces the manual effort needed to collect and process data, allowing you to focus on analysis and decision-making.
- Consistency: Automated processes ensure that data retrieval occurs at regular intervals, providing up-to-date insights without delay.
- Customization: Scripts can be tailored to retrieve specific data points based on campaign requirements and reporting needs.
Summary of Data Fields
Field Name | Description |
---|---|
campaign_name | The name of the advertising campaign |
impressions | The number of times the ad was displayed |
clicks | The number of times the ad was clicked |
spend | The total amount spent on the campaign |
Optimizing Your Facebook Ads API Calls for Faster Results
When working with the Facebook Ads API, ensuring that your requests are optimized can greatly improve performance. This not only saves time but also reduces the load on the API, resulting in more efficient data retrieval. Proper management of your API calls can make a significant difference in terms of response time and overall user experience.
Understanding the nuances of making efficient requests, including reducing the number of calls and handling them in bulk, can lead to faster processing times. Several key strategies can help in streamlining your interactions with the Facebook Ads API.
Best Practices for Optimizing API Calls
- Use Batch Requests: Rather than making multiple individual requests, batch them into a single call to reduce overhead and latency.
- Specify Fields: Always specify the exact fields you need in the API request, avoiding unnecessary data fetching.
- Use Time Ranges: Filter data by date or time range to limit the amount of information returned and improve speed.
Advanced Techniques for Performance Gains
- Leverage Caching: Store commonly requested data locally to avoid making the same requests repeatedly.
- Asynchronous Requests: Implement async requests where possible to parallelize API calls and prevent delays.
- Utilize Long-Lived Access Tokens: Reducing the frequency of token refresh requests helps save time and reduces the load on the system.
Key Metrics for Performance Monitoring
Metric | Description |
---|---|
API Latency | Measures the delay between sending a request and receiving a response from the API. |
Request Failure Rate | Tracks the number of failed API requests, indicating potential issues with the request or data. |
Data Throughput | Refers to the volume of data retrieved or sent in a given time period, influencing the efficiency of the API calls. |
Important: Always test different configurations of your API requests to determine the best balance between speed and data accuracy for your needs.