Facebook Lead Ads Api Example Php
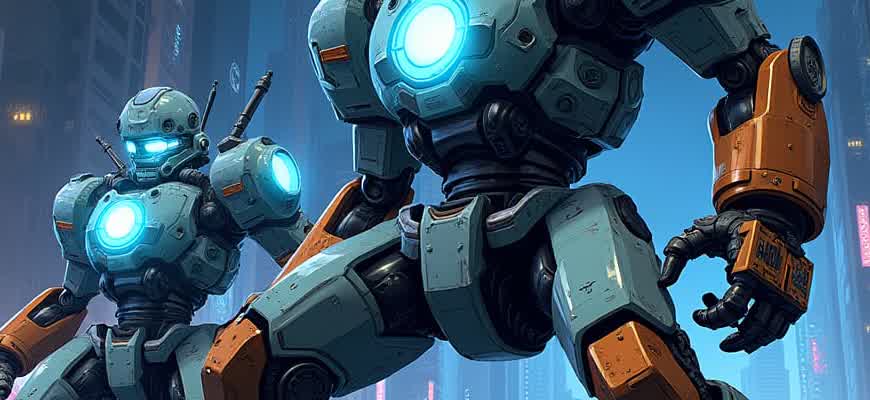
Integrating Facebook Lead Ads into your website using PHP provides an efficient way to capture leads directly from the platform. This integration allows you to automate data collection and improve your lead generation process. In this guide, we will walk through the process of setting up and using the Facebook Lead Ads API with PHP, providing a step-by-step approach for developers.
Prerequisites: Before proceeding, make sure you have the following:
- A Facebook Developer Account
- Facebook App with permissions to access the Lead Ads API
- PHP environment with cURL and JSON support
- Access to a Facebook Page and Lead Form
The next step involves setting up the API connection and making requests to retrieve lead data. Below is an example of how to authenticate and fetch lead data using PHP:
Note: Ensure your access token has the required permissions, such as ads_management and leads_retrieval, to fetch lead information.
Here’s an example of how to get lead data:
$accessToken, ]; $response = file_get_contents($endpoint . '?' . http_build_query($params)); $leads = json_decode($response, true);
Response Data:
Lead ID | Field Name | Field Value |
---|---|---|
12345 | Full Name | John Doe |
12345 | [email protected] |
Working with Facebook Lead Ads API using PHP
Integrating Facebook Lead Ads with PHP allows developers to automate the process of collecting leads directly from Facebook Ads. This can significantly enhance marketing efforts by seamlessly transferring user data into a CRM or another database. The Facebook Lead Ads API enables businesses to retrieve, manage, and store leads from forms created on Facebook's platform.
In this example, we will explore how to set up a basic PHP script to retrieve lead information using the Facebook API. This will involve connecting to the API, authenticating using an access token, and handling responses to capture lead data efficiently.
Steps for Retrieving Lead Data
To start working with the Facebook Lead Ads API, you need to follow these essential steps:
- Set Up Facebook Developer Account: You must have a Facebook Developer account to create an app and generate the necessary credentials.
- Obtain an Access Token: The API requires a valid access token to authenticate your requests. This token can be generated through the Facebook Graph API Explorer or via OAuth.
- Retrieve Form Leads: Use the API endpoint to fetch the data submitted by users through lead generation forms.
Example Code for Retrieving Leads
Below is an example of how to implement the process in PHP:
"; echo "Field 1: " . $lead['field_data'][0]['values'][0] . "
"; } ?>
Important Notes
Make sure that your Facebook App is set up correctly to access lead data. The user must have the necessary permissions, such as "leads_retrieval" permission, for your app to access the lead information.
Handling the Response
When you make a request to the API, the response will be in JSON format. You will need to parse this JSON data to access specific fields of the lead forms.
Field | Description |
---|---|
id | Unique identifier for the lead submission |
field_data | Contains an array of fields and the user's submitted values |
Configuring Your Facebook App for Lead Ads API Integration
Before integrating the Facebook Lead Ads API into your system, it's essential to correctly configure your Facebook application. This process includes setting up the required permissions, generating an access token, and linking your Facebook app with the necessary ad account. Proper configuration ensures smooth data exchange between your app and Facebook's ad platform, enabling you to efficiently manage leads generated from ads.
To begin, you need to have a Facebook Developer account. After creating your account and setting up the app, you can start the configuration process. Below are the key steps for setting up your Facebook app for API access:
Steps to Set Up Your App
- Create a Facebook Developer Account: If you haven't already, visit the Facebook Developer portal to create your account.
- Create a New App: Once logged in, create a new app under the "My Apps" section.
- Set Up App Permissions: Navigate to the 'App Review' section and ensure you request the appropriate permissions such as ads_management and leads_retrieval.
- Generate Access Token: In the "Tools" section, use the Access Token Tool to generate a token with the necessary permissions.
- Link Facebook Ad Account: Connect your ad account by linking it to the app settings in the Facebook Developer dashboard.
Important Information
Ensure that your app has been approved for the required permissions by Facebook before using the API in a production environment.
App Settings Table
Setting | Description |
---|---|
App ID | Unique identifier for your app, needed to authenticate requests. |
App Secret | Secret key used to authenticate your app and secure data exchanges. |
Access Token | Token used to authenticate API calls and retrieve data from the platform. |
After completing these steps, your Facebook app will be ready for Lead Ads API integration, allowing you to efficiently retrieve and manage leads generated through Facebook ads.
How to Authenticate Using Facebook Lead Ads API in PHP
When integrating the Facebook Lead Ads API in your PHP application, the first step is to authenticate and gain access to the necessary data. Facebook uses OAuth 2.0 for secure authentication, requiring developers to acquire an access token. This token will grant permission to interact with the Lead Ads API on behalf of the Facebook user or page.
To authenticate, you need to follow a few key steps. This involves obtaining the correct permissions from the user, using the Facebook SDK for PHP, and managing access tokens securely. Below is a detailed guide on how to implement authentication effectively using PHP.
Step-by-Step Authentication Process
- Create a Facebook App: You need to create a Facebook App in the Facebook Developer Dashboard to obtain your app's credentials such as App ID and App Secret.
- Obtain User Access Token: Using the Facebook SDK for PHP, prompt the user to log in to their Facebook account and grant your app permission to access their Lead Ads data.
- Exchange Code for Token: Once the user logs in, Facebook will redirect back to your application with a code. This code can be exchanged for a long-lived access token using the Graph API.
- Store Token Securely: It's critical to securely store the access token for future API requests. Use server-side storage like a database to keep the token safe.
Example PHP Code
'your-app-id', 'app_secret' => 'your-app-secret', 'default_graph_version' => 'v15.0', ]); $helper = $fb->getRedirectLoginHelper(); $permissions = ['email', 'ads_management']; // Permissions needed $loginUrl = $helper->getLoginUrl('https://your-redirect-url.com/', $permissions); echo 'Log in with Facebook!'; ?>
Important: Make sure to use the proper redirect URL and set the correct permissions to access the Lead Ads API.
Common Issues During Authentication
Issue | Solution |
---|---|
Invalid App ID or App Secret | Double-check your credentials in the Facebook Developer Dashboard. |
Access Token Expired | Request a new access token using the refresh process. |
Missing Permissions | Ensure you are requesting the correct permissions, such as 'ads_management' and 'leads_retrieval'. |
Retrieving Lead Data with Facebook Lead Ads API: PHP Example
When working with Facebook Lead Ads, one of the primary tasks is to access the data collected through forms. This can be done by leveraging the Facebook Lead Ads API, which allows businesses to retrieve leads in an automated and efficient manner. In this example, we will discuss how to use PHP to fetch the lead information, making the process smooth and scalable for developers.
To successfully retrieve lead data from Facebook, you need to authenticate and connect with Facebook's Graph API. This involves setting up an app on the Facebook Developer platform, obtaining an access token, and using it to make requests to the API endpoints. Below is a step-by-step guide to implement this in PHP.
Steps for Retrieving Leads in PHP
- First, ensure you have the Facebook SDK for PHP installed in your project. You can install it using Composer:
- Set up your app credentials from the Facebook Developer portal. You will need the App ID, App Secret, and a User Access Token.
- Use the access token to authenticate your requests to the API. Below is an example of how to initiate the API call in PHP:
- Once you fetch the data, you can process the lead data as required, for example, storing it in a database or displaying it on your platform.
composer require facebook/graph-sdk
$fb = new \Facebook\Facebook([ 'app_id' => 'YOUR_APP_ID', 'app_secret' => 'YOUR_APP_SECRET', 'default_graph_version' => 'v11.0', ]); try { $response = $fb->get('/{page-id}/leadgen_forms?access_token={access-token}'); $lead_data = $response->getDecodedBody(); } catch(Facebook\Exceptions\FacebookResponseException $e) { echo 'Error: ' . $e->getMessage(); }
Sample Lead Data
The response from the API will contain details about the leads collected through the forms. A sample structure of the retrieved data might look like the following:
Field Name | Description |
---|---|
lead_id | Unique identifier for each lead |
Email address submitted by the user | |
full_name | Full name of the lead |
phone_number | Phone number (if provided) |
Note: Always ensure that you handle user data responsibly and in accordance with GDPR or other relevant privacy regulations.
Handling Facebook Lead Ads Webhooks with PHP
When integrating Facebook Lead Ads with your PHP application, it's crucial to set up an efficient system to handle incoming webhook notifications. Facebook uses webhooks to send real-time data whenever a user submits a lead form. In this process, PHP plays a central role in parsing, verifying, and processing the incoming data to ensure that the leads are captured properly and securely.
In this guide, we will focus on how to configure PHP to manage these webhooks, ensuring that the data received from Facebook is validated and processed as needed. This includes setting up a webhook listener and responding to Facebook’s verification request to confirm that your server is ready to handle the notifications.
Steps to Implement Webhook Handling
- Set up a Webhook URL: Create a PHP script that will serve as your webhook endpoint. This URL will be where Facebook sends the lead data.
- Verify the Webhook: Facebook requires you to verify your webhook subscription. You must handle the verification request by responding with the `hub.challenge` parameter sent by Facebook.
- Parse Lead Data: Once the webhook is set up, you’ll need to parse the incoming lead data, which will typically be in JSON format. You should extract the relevant information and store it securely.
- Handle Errors: Implement error handling to manage unexpected issues, such as missing data or invalid payloads.
To verify your webhook, you must check that the 'hub.verify_token' matches the value you’ve configured and return the 'hub.challenge' to confirm the subscription.
Example Code for Facebook Webhook in PHP
The following is an example of a simple PHP script to handle Facebook's webhook verification and process incoming lead data:
Field | Value |
---|---|
Method | GET or POST |
Verification Token | Your custom token |
Lead ID | From the incoming POST data |
Extracting and Storing Lead Information from Facebook in Your Database
When dealing with lead data from Facebook, the first crucial step is to properly extract the data through Facebook's API. By using Facebook's Lead Ads API, you can retrieve detailed information about the leads generated from your campaigns. This data typically includes user inputs such as name, email, phone number, and other custom fields you set up in your lead forms.
Once the data is retrieved, it needs to be parsed and securely stored in your database for further use. Parsing the data involves extracting each lead’s details and organizing them in a structured format that aligns with your database schema. This ensures that you can efficiently access and process the data for future actions such as follow-up emails, CRM updates, or analytics.
Steps to Parse and Store Data
- Authenticate with Facebook’s Graph API using an access token.
- Request lead data for a specific ad form or campaign.
- Parse the JSON response to extract relevant information (e.g., name, email, custom fields).
- Map the data to the appropriate columns in your database (e.g., leads table).
- Store the data securely in the database, ensuring proper sanitization and validation.
Important: Ensure that you follow Facebook’s policies for handling lead data, especially regarding user privacy and data protection.
Sample Database Schema
Field Name | Data Type |
---|---|
lead_id | INT |
name | VARCHAR(255) |
VARCHAR(255) | |
phone | VARCHAR(50) |
custom_field | TEXT |
Tip: It's essential to store lead data with proper indexing for faster queries, especially if you are handling a large volume of leads.
Managing Lead Data: Filtering, Sorting, and Exporting with PHP
When working with lead generation campaigns through Facebook Ads, effectively managing incoming lead data is essential. Using PHP, developers can filter, sort, and export this data to streamline the marketing efforts. These operations allow you to quickly organize leads based on specific criteria, ensuring that your team can follow up with the most relevant prospects first.
PHP offers a variety of tools for interacting with lead data, including database queries and file exports. By applying filters to lead records, such as date ranges or specific campaign attributes, you can target high-value leads. Sorting allows you to prioritize them based on relevant characteristics like engagement level or purchase intent, while exporting ensures that you can share and analyze data efficiently.
Filtering Lead Data
To filter incoming lead data, developers can use PHP to process the JSON responses from Facebook's Lead Ads API. This can involve checking certain fields such as lead creation date, lead source, or custom attributes defined in the form. Below is an example of how to filter leads that were submitted after a specific date:
$filteredLeads = array_filter($leads, function($lead) {
return strtotime($lead['created_time']) > strtotime('2023-01-01');
});
This function checks the created_time field for each lead and includes only those that meet the date condition.
Sorting Lead Data
Once you have filtered your data, sorting can help prioritize which leads to follow up with first. Sorting by specific fields such as first_name, last_name, or email helps organize the leads. For example, to sort by last name in ascending order, you can use the following code:
usort($filteredLeads, function($a, $b) {
return strcmp($a['last_name'], $b['last_name']);
});
This will order the leads alphabetically by last name, allowing you to sort and organize them efficiently.
Exporting Lead Data
Exporting the filtered and sorted lead data is a critical step in the lead management process. PHP provides various methods for exporting data to different file formats. A common approach is exporting leads to a CSV file, which can be opened in Excel for further analysis. Here is an example of how to create a CSV file from the lead data:
$csvFile = fopen('leads.csv', 'w');
fputcsv($csvFile, ['First Name', 'Last Name', 'Email', 'Phone']); // Column headers
foreach ($filteredLeads as $lead) {
fputcsv($csvFile, [$lead['first_name'], $lead['last_name'], $lead['email'], $lead['phone']]);
}
fclose($csvFile);
This code writes the filtered leads to a CSV file with column headers and lead details. Once exported, you can open the file in any spreadsheet software for easy analysis.
Important Considerations
When handling lead data, always ensure compliance with data protection regulations such as GDPR. Safeguard personal information and ensure it is stored and shared securely.
Conclusion
With PHP, managing Facebook Lead Ads data is both efficient and flexible. By filtering, sorting, and exporting lead data, you can optimize your marketing efforts and ensure that the most valuable leads are followed up promptly. The ability to export the data in a format like CSV further enhances collaboration and analysis, making it easier to track your campaign performance.
Optimizing API Calls: Reducing Latency in Facebook Lead Ads Integration
Integrating Facebook Lead Ads into a system can significantly improve lead generation, but the efficiency of the API calls plays a critical role in overall performance. Minimizing latency in API interactions ensures a smoother user experience and faster processing of submitted lead data. Reducing response time is crucial for both scalability and user satisfaction, especially when dealing with large volumes of leads. Implementing proper strategies to optimize these interactions will make a significant difference in system efficiency.
In this context, the focus should be on minimizing network delays, optimizing data handling, and ensuring smooth communication between your server and Facebook's API. Several methods, such as batching requests and caching responses, can help reduce the time spent waiting for the API’s response. Below are some practical approaches to enhancing the performance of Facebook Lead Ads integration.
Best Practices to Optimize API Calls
- Batching Requests: Instead of sending individual requests for each lead, consider batching multiple leads into a single API request. This reduces the number of network round trips, which can significantly cut down on latency.
- Utilizing Webhooks: Facebook offers webhooks that notify your system when a lead is submitted. This reduces the need for polling, which can be resource-intensive and slow. By using webhooks, you can retrieve leads almost instantly when they are submitted.
- Data Caching: Cache frequently accessed data, such as campaign information or form structures. This reduces the number of calls made to Facebook’s servers, as you can retrieve the cached data locally without needing to query the API every time.
- Parallel API Calls: When handling multiple lead forms, initiate parallel API calls where possible. This allows your system to process several requests simultaneously, reducing overall latency.
Considerations for Reducing Response Times
- Efficient Data Processing: Process the data on your server asynchronously, ensuring that API calls do not block other essential operations.
- Optimizing JSON Parsing: When receiving responses from Facebook's API, make sure to optimize how your system parses and processes JSON data. Poor parsing routines can add significant overhead and increase response times.
- API Rate Limits: Ensure that your application respects Facebook's API rate limits to avoid unnecessary delays caused by throttling. Exceeding these limits can cause your requests to be queued or denied, leading to slower processing times.
Example of Optimized API Call Strategy
Method | Impact on Latency | Notes |
---|---|---|
Batch Requests | Reduces API calls and network round trips | Helps reduce delays for high-volume lead forms |
Webhooks | Instant lead data delivery | Reduces the need for frequent polling |
Data Caching | Decreases the number of API calls | Speeds up response times for repeated data |
Effective API call optimization can lead to better user experience, faster data processing, and improved overall system efficiency. By implementing the strategies mentioned above, you can ensure your Facebook Lead Ads integration is both performant and scalable.
Troubleshooting Common Problems with Facebook Lead Ads API Using PHP
Integrating Facebook Lead Ads API with PHP can sometimes lead to various issues that require attention. Proper troubleshooting is crucial to ensure smooth operation and accurate data retrieval from the leads generated through Facebook campaigns. In this guide, we’ll go over some common issues developers face when working with the API and how to solve them efficiently.
From authentication errors to data inconsistencies, developers may encounter a range of problems during integration. These issues can stem from incorrect API usage, missing permissions, or improper setup of your PHP environment. By understanding how to identify and resolve these challenges, you can streamline the development process and improve the overall performance of your integration.
Authentication Errors
One of the most common issues is related to authentication when accessing the Facebook Lead Ads API. These errors typically occur when your access token is expired, invalid, or lacks the necessary permissions. Make sure the following:
- Ensure your access token has not expired by regenerating it regularly or using long-lived tokens.
- Verify that the token has the correct permissions to access leads, such as ads_management or leads_retrieval.
- Check if the token is associated with the correct Facebook App and Facebook Ad Account.
Tip: Always handle token expiration gracefully in your PHP code, refreshing tokens when necessary to avoid interruptions in lead retrieval.
Data Retrieval Issues
Another common problem involves retrieving lead data from Facebook. If the data seems incomplete or missing, ensure the following:
- Confirm that you are using the correct lead form ID and ad account ID in your API requests.
- Check for data delays as Facebook might take time to process new leads.
- Review the API response to identify any error messages that might indicate a problem with your request.
Error Code | Possible Cause | Solution |
---|---|---|
102 | Invalid Access Token | Regenerate and validate the access token |
104 | Insufficient Permissions | Ensure correct permissions for the API call |
Note: When debugging lead retrieval issues, consider using Facebook's Graph API Explorer to test your requests before implementing them in your PHP code.