Facebook Banner Ads Test Id In Android
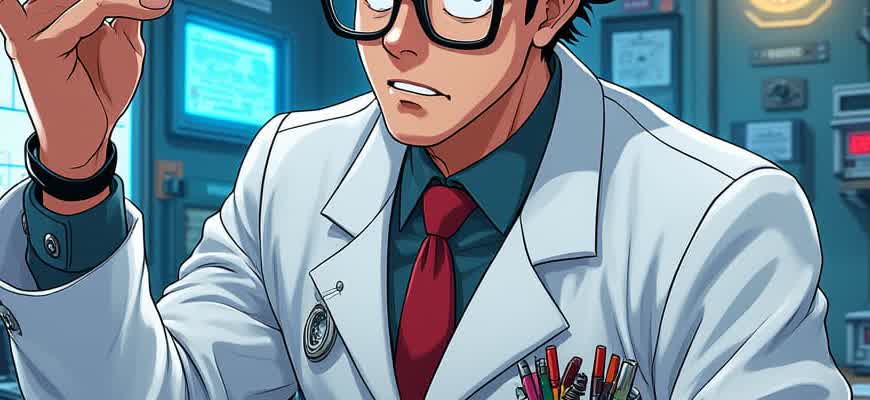
Testing Facebook Banner Ads in Android apps requires careful configuration and proper identification of ad units. One of the first steps is understanding how to implement and validate the Facebook Ads ID used for banner ad displays.
There are several key points to consider during this process:
- Ensure proper SDK integration.
- Check for the correct placement of banner ads in the Android layout.
- Test using valid and unique Ad IDs.
Testing with real-time data allows for accurate tracking of performance and behavior under actual conditions.
Steps for validating Facebook Banner Ads ID:
- Open the Android Studio project.
- Implement the Facebook Audience Network SDK.
- Insert the Ad ID into the proper XML layout or code section.
- Run the app on a physical device to test the banner ad display.
Key considerations when testing:
Factor | Details |
---|---|
Ad Load Time | Monitor how quickly the ad loads on different network conditions. |
Performance Impact | Check if the banner ad affects the app’s performance or responsiveness. |
Understanding the Role of Test Ids in Facebook Banner Ads
Test identifiers (Test Ids) are an essential part of the process when integrating Facebook banner ads in Android applications. These unique IDs allow developers to test ads in a safe environment without serving real advertisements to users. By using test IDs, developers can simulate ad loading, display, and interaction behaviors without incurring any costs or violating Facebook's policies. This makes it easier to ensure that the ad integration works seamlessly within the app before going live.
When implementing Facebook banner ads, it is crucial to use the correct test ID to avoid errors in the ad display and functionality. Incorrect or expired test IDs can lead to failed ad loads, incorrect ad behavior, or even violations of Facebook’s ad policies. In addition to providing a safe testing environment, these IDs help developers fine-tune ad placement, size, and performance within their Android applications.
Key Benefits of Using Test Ids in Facebook Banner Ads
- Safe Testing: Test IDs allow developers to evaluate ad functionality without using live advertisements, avoiding unnecessary costs and errors.
- Compliance with Policies: Using Facebook's provided test IDs ensures that developers follow their ad integration guidelines and do not display live ads during testing.
- Streamlined Debugging: Test ads help identify integration issues in a controlled setting, making it easier to resolve technical problems before launching to users.
Process of Using Test Ids for Banner Ads
- Generate a test ad ID from the Facebook Developer portal.
- Integrate the test ID into the ad loading code of your Android application.
- Verify that the ad loads correctly and interacts as expected in the app.
- Replace the test ID with the live ad ID once you’re ready for production.
Important: Always use the official test ad IDs provided by Facebook to avoid issues with ad display and to ensure compliance with platform policies.
Common Issues with Test Ids
Issue | Solution |
---|---|
Test ID Not Loading Ads | Check if the test ID is valid and ensure that the ad placement in the code is correct. |
Incorrect Ad Behavior | Review the implementation to make sure the ad format matches the ad unit type selected in the Facebook Developer portal. |
Expired Test ID | Generate a new test ID from the Facebook Developer portal and update the code accordingly. |
Setting Up Test ID for Banner Ads in Your Android App
When integrating banner ads into your Android app, it's crucial to set up test identifiers to ensure proper functionality during development. This step prevents accidental clicks or impressions from being recorded on live ad campaigns, which could violate the terms of service. Additionally, using a test ID helps to check how your app's ad placement appears on different screen sizes and resolutions without affecting your account's statistics.
In this guide, we'll walk through the process of configuring a test ID for banner ads in your Android project. Following these steps ensures that you're working within best practices for app development and advertising integration.
Steps to Configure Test ID for Banner Ads
- Go to the Facebook Audience Network website and log in to your developer account.
- Navigate to the Monetization Manager section.
- Find the Ad Placements tab and select the banner ad type.
- Generate a Test ID under the Test Devices section.
- Copy the generated Test ID for use in your Android app.
Implementing Test ID in Your Android Project
- Add the necessary dependencies for the Facebook Audience Network SDK in your build.gradle file.
- In your MainActivity.java file, initialize the AdSettings class and set the test ID:
- Load the banner ad by specifying the test ID in the ad view configuration:
- Test the banner ad by running the app on a physical device.
AdSettings.addTestDevice("YOUR_TEST_DEVICE_ID");
AdView adView = new AdView(this);
adView.setAdUnitId("YOUR_TEST_AD_UNIT_ID");
adView.loadAd(new AdRequest.Builder().build());
Ensure that you're using only the test IDs during the development phase. Once you're ready for production, replace the test ID with the live ad unit ID provided by Facebook.
Important Notes
Step | Details |
---|---|
Test ID | Used to prevent accidental clicks and to ensure your app is functioning correctly with banner ads during development. |
Live Ad Unit ID | To be used when your app is ready for public release. Test IDs should never be used in production. |
Integrating Facebook Banner Ads with Your Android Application Using Test Id
To effectively test Facebook banner ads within your Android application, using a test ID is essential to avoid serving live ads during development. A test ID allows developers to preview how ads will appear without violating Facebook’s ad policies or risking improper ad serving. This guide will show you the steps to integrate banner ads using a test ID into your Android app.
Follow the outlined steps below to get started with Facebook banner ads integration and testing. Ensure you have a Facebook Developer account and have already set up your app within the Facebook Audience Network.
Steps to Integrate Facebook Banner Ads Using Test ID
- First, ensure that you have added the necessary dependencies for Facebook Audience Network in your project’s build.gradle file:
- In your app's MainActivity, initialize the Facebook Audience Network SDK:
- Create a Banner Ad instance using the test ID provided by Facebook for development:
- Next, set up the banner ad view in your layout file:
- In the activity, load the ad using the test ID:
implementation 'com.facebook.android:audience-network-sdk:6.5.0'
AudienceNetworkAds.initialize(this);
String testBannerAdId = "YOUR_TEST_BANNER_AD_ID";
<com.facebook.ads.AdView android:id="@+id/banner_ad" android:layout_width="match_parent" android:layout_height="wrap_content" />
AdView adView = findViewById(R.id.banner_ad); AdSize adSize = AdSize.BANNER_HEIGHT_50; adView.setAdSize(adSize); adView.setAdUnitId(testBannerAdId); adView.loadAd();
Important Considerations
Always use the test IDs provided by Facebook to avoid any violations of their ad policies during the development phase.
Testing Banner Ads
Once the test ID is integrated into your app, you can run it on an emulator or physical device to see how the ad appears. It is important to ensure that the ad renders properly without any errors. Below is a table summarizing the key steps and their expected outcomes during the integration process:
Step | Expected Outcome |
---|---|
Add dependencies | Dependencies for Facebook Audience Network SDK are successfully added to the project. |
Initialize SDK | The SDK is initialized and ready for ad loading. |
Set test ID | The test ID is correctly assigned to the banner ad view. |
Load Ad | The banner ad is loaded with the test ID and displayed on the screen. |
Common Errors When Using Test Identifiers in Facebook Banner Ads
When implementing Facebook Banner Ads in Android applications, developers often use test identifiers to ensure that their ad integration works correctly without displaying real ads to users. However, there are several common mistakes that can occur when working with these identifiers. These errors can cause unexpected behavior, such as ads not loading or showing irrelevant content during testing.
Understanding these common issues is crucial for optimizing ad implementations and ensuring that the testing process is as effective as possible. Below are some of the frequent errors developers encounter when using test IDs with Facebook Banner Ads.
1. Using Incorrect or Expired Test IDs
One of the most common errors is using outdated or incorrect test IDs. Facebook periodically updates their testing IDs, and using an expired one can result in failed ad requests or improper behavior during testing.
Important: Always check Facebook's official documentation for the most recent test IDs to avoid issues with your ad requests.
- Check the ID format carefully.
- Ensure you are using the correct ID for banner ads specifically, not for other ad types.
- Verify that the ID has not expired by consulting the latest information on the Facebook developer site.
2. Not Handling Test Ad Responses Properly
Another common mistake is failing to correctly handle the responses from the test IDs. This can include not displaying ads when they should appear or showing ads incorrectly on the screen.
Important: Always ensure you have implemented proper error handling for the ad loading process, especially when testing.
- Implement event listeners to monitor ad load success or failure.
- Check for the correct display parameters (size, position) when testing banner ads.
- Use logging to track ad requests and responses during development.
3. Misconfiguration of Ad Placement or Size
Developers sometimes misconfigure the ad placement or size when using test identifiers. This can cause the ad to appear in the wrong location or with incorrect dimensions, making testing less effective.
Possible Configuration Errors | Effect on Testing |
---|---|
Incorrect ad placement (e.g., not properly setting the container view) | Ad may appear outside the expected area of the screen or not appear at all. |
Incorrect ad size (e.g., using a full-screen ID for a banner ad) | The ad may appear too large or too small for the screen. |
Important: Double-check the ad size and placement code to ensure proper configuration during testing.
How to Verify Facebook Banner Ads Display Correctly Using a Test ID in Android
Ensuring that Facebook banner ads appear as intended in your Android application is crucial for providing a seamless user experience. One of the most effective ways to verify this is by using a Facebook Test ID. This allows developers to test ads without showing them to actual users. In this process, you can identify and resolve any potential issues with ad placement, loading time, and overall display quality before pushing your app live.
To verify your Facebook banner ads, follow these straightforward steps to check if the ads are functioning correctly on your Android app.
Steps to Verify Facebook Banner Ads Using Test ID
- First, create a test ad ID on Facebook’s platform and integrate it into your Android project.
- Ensure that the ad unit is properly linked to the layout where the banner should appear.
- Implement Facebook's SDK for testing purposes and configure your app to fetch ads based on the test ID.
Once the setup is complete, use the following methods to verify the functionality:
- Launch the app in testing mode on your Android device.
- Check that the banner ad is visible, aligned properly within the app's UI, and loaded without delay.
- Confirm that no errors or blank spaces are present where the ad should be shown.
Important: Test IDs ensure ads are displayed only in test environments and not to end users. Make sure to replace the test ID with the live ad ID before going public.
Table for Ad Verification Status
Test Criteria | Status |
---|---|
Banner Appears | Yes/No |
Ad Alignment | Correct/Incorrect |
Ad Load Time | Fast/Slow |
Best Practices for Testing Banner Ads in Your Android Application
Testing banner ads in your Android app is a critical step to ensure smooth user experience and to optimize monetization. Without proper testing, you might face issues like poor ad loading performance or an incorrect display format, which can lead to a bad user experience. To avoid these issues, developers need to follow best practices when testing banner ads in their Android application.
In this guide, we will outline key strategies for testing banner ads, including different testing methods and tools. By following these tips, you can improve your ad performance and make sure your app works as intended across different devices.
Key Testing Methods for Banner Ads
- Use Test Ads: Always start with test ads during development to avoid generating real impressions. Facebook and Google provide test ad units for this purpose.
- Test on Multiple Devices: Banner ads might render differently depending on the device's screen size, resolution, or operating system version. Test on a variety of devices to ensure compatibility.
- Use Different Ad Sizes: Test various ad formats (e.g., standard banners, full banners) to see how they perform and whether they fit well within your app's layout.
- Check for Proper Ad Placement: Ensure that ads do not overlap with important UI elements and do not negatively impact the app's usability.
Tools to Help with Banner Ads Testing
- Facebook Audience Network (FAN) Test Device: Use Facebook’s own test devices to simulate banner ad behavior on real devices without the risk of generating real ad impressions.
- AdMob Test Ads: Use Google AdMob's built-in tools for testing ads in your app without affecting actual revenue.
- Android Emulator: For initial testing, Android emulators can simulate different device configurations, screen sizes, and ad placements.
Important Considerations
Testing ads with real user data during development is not recommended. Always use the provided test ad units to prevent accidental clicks and inaccurate analytics.
Sample Test Scenarios for Banner Ads
Test Scenario | Description | Expected Outcome |
---|---|---|
Banner Ad on Different Screen Sizes | Test banner placement on phones, tablets, and devices with varied screen resolutions. | Banner should load without distortion and be properly aligned with the layout. |
Ad Visibility | Ensure ads are visible in all app states (e.g., portrait, landscape, background). | Ads should remain visible and appropriately sized under all conditions. |
Ad Click Behavior | Verify that clicking on an ad opens the expected URL or action. | Ad should correctly redirect to the target action, whether it’s an external URL or in-app action. |
How to Replace Test Id with Real Ad Ids in Your Android App
When you are integrating Facebook ads into your Android application, it's essential to replace the test ad ids with real ad ids before going live. This ensures that users see real ads and your app generates revenue as intended. Initially, you may use test ids for debugging and testing purposes, but real ad ids should be implemented for actual app usage. The process is straightforward but requires attention to detail to avoid mistakes.
Here are the steps to replace test ids with real ad ids in your Android app:
Steps to Replace Test Id with Real Ad Ids
- Access Your Facebook Ad Manager: Navigate to the Facebook Ads Manager to obtain the real ad ids for your banners, interstitials, or other ad types.
- Locate Test Ad Ids in Code: In your Android project, search for the places where test ad ids are being used in your ad initialization code.
- Replace with Real Ad Ids: Replace the test ids with the actual ad ids that you got from Facebook Ads Manager.
- Double Check Your Code: Make sure that you have replaced every occurrence of the test ids to prevent any accidental use of them in a production environment.
It's important to replace all test ids with real ones before releasing your app to ensure you are not violating Facebook's ad policies.
Example Code for Replacing Test Ad Id
Below is an example to demonstrate how to replace a test ad id with a real ad id in your Android app code:
// Test Ad Id
String testAdId = "YOUR_TEST_AD_ID";
// Replace with Real Ad Id
String realAdId = "YOUR_REAL_AD_ID";
// Initialize AdView with Real Ad Id
AdView adView = new AdView(this);
adView.setAdUnitId(realAdId);
Additional Tips
- Check for Errors: Before publishing, test the ads in the production environment to make sure they are displaying correctly.
- Monitor Ad Performance: After switching to real ad ids, regularly check your ad performance through Facebook's reporting tools.
- Stay Updated: Facebook occasionally updates its ad policy or SDK, so ensure your app is always using the latest versions.
Debugging Tips for Facebook Banner Ads with Test Ids on Android
When working with Facebook Banner Ads on Android, it's crucial to ensure that the integration is smooth and functioning correctly. Using Test IDs is an essential step for verifying ad delivery without violating Facebook's ad policies. In this guide, we will explore several tips to help you debug the process effectively and resolve common issues with test IDs on Android devices.
Testing Facebook Ads in your Android application can present a few challenges. From incorrect initialization to ad loading failures, it's important to go through a structured debugging process. Below are some tips and best practices to troubleshoot Facebook banner ads with Test IDs and improve their reliability in your app.
Key Debugging Strategies
- Ensure Test ID is Correctly Configured: Always double-check that you are using a valid Facebook test ID for banners. You can find these IDs in Facebook's developer documentation or use the ID specifically provided for testing purposes.
- Initialize the Ad Properly: Use the correct initialization methods for Facebook SDK to ensure the banner ads are loaded before being shown on the screen.
- Check Permissions and Network Connectivity: Ensure your app has the necessary permissions and the device is connected to the internet for ad fetching.
- Monitor Logcat for Errors: Use Logcat to track any issues related to banner ad loading. Look for error messages that could indicate initialization or network-related issues.
Common Issues and How to Resolve Them
- Ad Not Loading:
- Verify if your ad request is successful and ensure that there is an active internet connection.
- Ensure that Facebook’s SDK has been properly initialized in the application.
- Incorrect Ad Display:
- Ensure the layout of the banner is set correctly within your activity or fragment.
- Check if the ad is sized properly and fits within the designated space in the UI.
- Test ID Not Working:
- Confirm the Test ID you are using is valid. Test ads may not show immediately, but they should eventually load.
- Make sure you're using the right Facebook Test ID associated with the app or test mode.
Important: Always test Facebook banner ads on actual devices, as emulators might not always reflect the behavior of real ads.
Debugging Tools and Resources
Tool | Description |
---|---|
Logcat | Use Android Logcat to check detailed logs for errors related to ad loading and display. |
Facebook Audience Network Dashboard | Monitor ad performance and troubleshoot any issues related to ad delivery directly from the Facebook dashboard. |
Test Ads from Facebook | Use Facebook's Test Ads feature to ensure your integration is working without displaying real ads to users. |